A look back: printing documentation on the Model 33
Explore computing history with this sample program to print one page at a time to a Model 33 terminal.
Technical writers have used computers to create documents for almost as long as we've had computers. If you can connect a computer to a printing device, it won't take long before someone will print a document with it.
An early computer terminal was the Teletype Model 33. There were several versions of the Model 33, but they all shared the common limitations: they were all typewriter-like devices that could only print in uppercase.
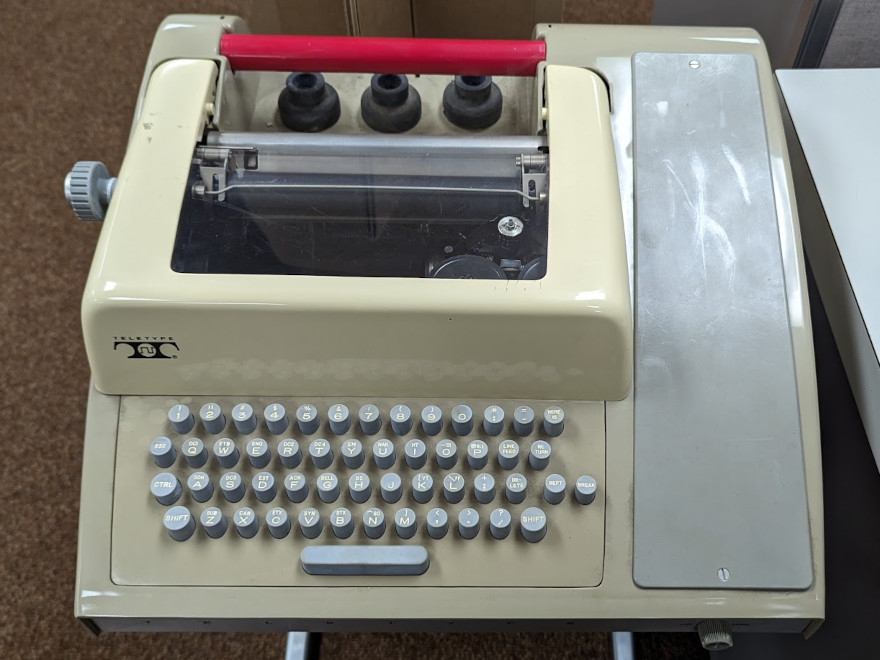
Printing to single sheet paper
Normally, the Model 33 printed its output to a long roll of paper. The paper fed into the back of the terminal, around a platen or roller, and past a ribbon and print head. However, users could insert single-sheet letter paper into the Model 33 to print on single sheet pages. For example, the Unix 2nd Edition manual describes a type
command that would send output one page at a time, then wait for the user to load a new sheet of paper and press Enter before printing the next page.
.P1
.po 9
.ds LH "6/12/72
.ds RH "TYPE (I)
.ds CF "- % -
.ds CH
.IP NAME 15
type -- type on single sheet paper
.IP SYNOPSIS
.I type
name1 ...
.IP DESCRIPTION
.I type
copys its input files to the standard output.
After every 66 lines, type stops and reads
the standard input for a new line character
before continuing.
This allows time for insertion of single
sheet paper.
.IP FILES
--
.IP "SEE ALSO"
--
.IP DIAGNOSTICS
--
.IP BUGS
--
.IP OWNER
dmr
The finished manual page, formatted with nroff -ms
:
6/12/72 TYPE (I)
NAME type ‐‐ type on single sheet paper
SYNOPSIS type name1 ...
DESCRIPTION type copys its input files to the standard
output. After every 66 lines, type stops and
reads the standard input for a new line char‐
acter before continuing. This allows time
for insertion of single sheet paper.
FILES ‐‐
SEE ALSO ‐‐
DIAGNOSTICS ‐‐
BUGS ‐‐
OWNER dmr
‐ 1 ‐
A simple version of the type command
The type command does not exist on modern Linux systems, because we have moved on from simple typewriter-like terminals to print documentation. However, the type program is a fairly simple program to demonstrate. Basically, the program does two things:
- Prints 66 lines from a file to the standard output; that's the Teletype terminal. Typical US typewriters, terminals, and similar line printers produced six lines per inch. With 11 inches on a US Letter size page, that's 66 lines per page.
- Reads from the standard input until it finds the Enter key. The Teletype keyboard is the standard input device.
Reading the standard input to look for an Enter key is a simple loop. Today, we would likely write this program using the C programming language, which was also available in Unix 2nd Edition. In fact, this sample code is written in the same style as original C.
This code uses the fgetc
function to read one character or key at a time until it finds the '\n'
("New line") code, which represents the Enter key:
newline()
{
int key;
do {
key = fgetc(stdin);
} while (key != '\n');
return 0;
}
Displaying 66 lines from a file can be done with a similar loop. This code sample reads one letter at a time with fgetc
, and counts how many '\n'
("New line") codes it finds. When it reads 66 lines, it calls the newline
function to pause execution until the user presses Enter:
type(in, out)
FILE *in;
FILE *out;
{
int ch;
int lines = 0;
ch = fgetc(in);
while (ch != EOF) {
fputc(ch, out);
if (ch == '\n') {
if (++lines == 66) {
newline();
lines = 0;
}
}
ch = fgetc(in);
}
return 0;
}
Putting it all together
With those functions to read a single key at a time, and to print 66 lines at a time, we can write this simple implementation of the type
program. This processes one or more files, printing one page at a time to the terminal. The user needs to load a new single sheet of paper for every page printed:
#include <stdio.h>
newline()
{
int key;
do {
key = fgetc(stdin);
} while (key != '\n');
return 0;
}
type(in, out)
FILE *in;
FILE *out;
{
int ch;
int lines = 0;
ch = fgetc(in);
while (ch != EOF) {
fputc(ch, out);
if (ch == '\n') {
if (++lines == 66) {
newline();
lines = 0;
}
}
ch = fgetc(in);
}
return 0;
}
main(argc, argv)
int argc;
char **argv;
{
FILE *pfile;
int i;
for (i = 1; i < argc; i++) {
pfile = fopen(argv[i], "r");
if (pfile) {
type(pfile, stdout);
fclose(pfile);
}
}
return 0;
}
Times have changed. Today, if we print at all, we print to a laser printer using a modern printer language like PostScript. More likely, we generate documentation in some electronic format, such as HTML, EPUB, or PDF. But it can be educational to take a look back at how technical writers created documentation in an era before the electronic print format.